はじめに
この記事では、Go言語を用いてRESTful APIを設計と構築する方法を紹介します。最終的には、シンプルで効率的なWebサービスを作るための基礎を学べます。また、認証やエラーハンドリング、テストの書き方についても触れます。
そのほかのWebアプリケーションの開発についてはこちらも参考にしてください
Goで高性能なウェブアプリを実装する:FasthttpとFiberの活用術

GoでのWebアプリケーション構築:Echoフレームワークの魅力とその詳細な活用法

Ginを使ってみる(インストールからHelloWorld表示まで)【Go言語】
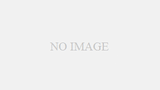
RESTful APIとは
RESTful APIは、REST (Representational State Transfer)というソフトウェアアーキテクチャのスタイルを用いて設計されたAPIです。このスタイルは、ウェブ全体の基本的な機能としてHTTPメソッド(GET、POST、PUT、DELETEなど)を使用することを特徴としています。
GoでRESTful APIを構築する
Goは効率的でシンプルな言語で、Webサービスの開発に適しています。特に、Goの標準パッケージである”net/http”は、HTTPサーバとクライアントの機能を提供し、RESTful APIの実装に便利です。
では、基本的なユーザ情報の取得と更新を行うAPIを作成してみましょう。
package main
import (
"encoding/json"
"net/http"
)
type User struct {
ID string `json:"id"`
Name string `json:"name"`
Age int `json:"age"`
}
var users = []User{
{"1", "John Doe", 25},
{"2", "Jane Doe", 24},
}
func getUser(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(users)
}
func main() {
http.HandleFunc("/users", getUser)
http.ListenAndServe(":8080", nil)
}
このコードを実行すると、localhostの8080ポートでHTTPサーバが起動します。そして、ブラウザからhttp://localhost:8080/users
にアクセスすると、ユーザのリストがJSON形式で表示されます。
次に、特定のユーザーを取得するAPIを追加しましょう。
//...
func getUser(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodGet {
id := r.URL.Query().Get("id")
for _, user := range users {
if user.ID == id {
json.NewEncoder(w).Encode(user)
return
}
}
w.WriteHeader(http.StatusNotFound)
} else {
w.WriteHeader(http.StatusMethodNotAllowed)
}
}
//...
このコードを実行してhttp://localhost:8080/users?id=1
にアクセスすると、IDが1のユーザー情報だけが取得できます。
さらに、ユーザー情報を更新するAPIも追加できます。そのためには、HTTPメソッドのPUTを使用します。
//...
import (
"io/ioutil"
//...
)
//...
func updateUser(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPut {
id := r.URL.Query().Get("id")
for i, user := range users {
if user.ID == id {
body, err := ioutil.ReadAll(r.Body)
if err != nil {
w.WriteHeader(http.StatusInternalServerError)
return
}
var updatedUser User
err = json.Unmarshal(body, &updatedUser)
if err != nil {
w.WriteHeader(http.StatusBadRequest)
return
}
users[i] = updatedUser
json.NewEncoder(w).Encode(updatedUser)
return
}
}
w.WriteHeader(http.StatusNotFound)
} else {
w.WriteHeader(http.StatusMethodNotAllowed)
}
}
func main() {
http.HandleFunc("/users", getUser)
http.HandleFunc("/users/update", updateUser)
http.ListenAndServe(":8080", nil)
}
上記のコードでは、HTTPメソッドがPUTの場合にユーザー情報を更新します。リクエストボディから新しいユーザ情報を読み取り、該当するユーザーの情報を更新します。そして更新したユーザーの情報をレスポンスとして返します。
以上のように、GoとHTTPパッケージを使用して、短時間で効率的にRESTful APIを構築できます。
テストの書き方
Goのテストは”testify”ライブラリを使用することで容易に書くことができます。以下はAPIのテストを書いた例です。
//...
import (
"github.com/stretchr/testify/assert"
"net/http/httptest"
"strings"
"testing"
)
func TestUpdateUser(t *testing.T) {
request := httptest.NewRequest(http.MethodPut, "/users/update?id=1", strings.NewReader(`{"id":"1", "name":"John Smith", "age":30}`))
responseRecorder := httptest.NewRecorder()
updateUser(responseRecorder, request)
assert.Equal(t, http.StatusOK, responseRecorder.Code)
user := User{}
err := json.NewDecoder(responseRecorder.Body).Decode(&user)
assert.NoError(t, err)
assert.Equal(t, "1", user.ID)
assert.Equal(t, "John Smith", user.Name)
assert.Equal(t, 30, user.Age)
}
このテストコードは、ユーザー情報の更新リクエストをシミュレートして、期待通りに更新されていることを確認します。
まとめ
Goの便利さと強力さを活用すれば、ほとんどのWebサービスを効率よく構築できます。ぜひこの機会に、Goを使用したWebサービスの開発を体験してみてください。
参考リンク
- Go公式ドキュメンテーション: https://golang.org/doc/
- Go net/httpパッケージ: https://golang.org/pkg/net/http/
- Testifyライブラリ: https://github.com/stretchr/testify